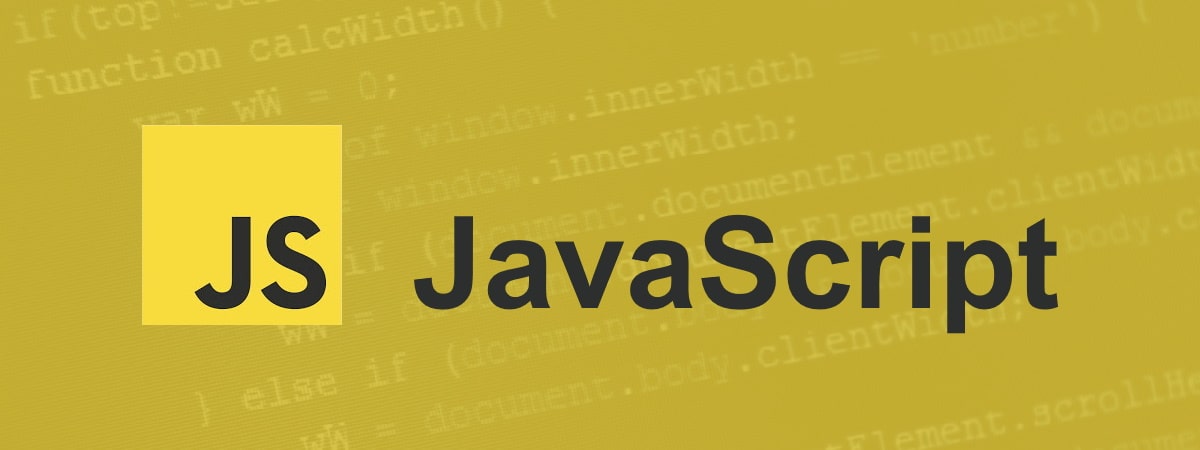
Cambiare il colore o la classe di un elemento HTML ciclicamente a tempo
Recentemente un collega mi ha chiesto un JavaScript per cambiare ciclicamente il colore si sfondo di un elemento HTML ogni N secondi.
Lo script seguente è una versione migliorata che supporta anche il cambiamento della classe CSS (sgart.assignValuesCyclically):
un esempio di utilizzo è il seguente:
dove l'esempio test 1 è richiamato passando un array di colori con un tempo di transizione di 2 secondi:
l'esempio test 2 è richiamato con un tempo di transizione di 2 secondi ma usa il set di colori predefinito:
l'esempio test 3 è richiamato passando un array di classi e con l'ultimo parametro impostato a true:
in questo caso non controllo solo il colore ma qualsiasi proprietà CSS.
L'ultimo esempio test 4 utilizza una diversa versione dello script che è la seguente (sgart.assignValuesCyclicallyEnhanced):
ed è richiamato passando un array di oggetti, dove, ogni oggetto contiene sia il colore (v) che il tempo di transizione (s):
Con le opportune modifiche è possibile gestire anche il cambio del source di un immagine o l'immagine di sfondo di un elemento HTML:
ovviamente l'array passato dovrà contenere i valori adeguati.
Il codice HTML usato per l'esempio è il seguente:
Lo script seguente è una versione migliorata che supporta anche il cambiamento della classe CSS (sgart.assignValuesCyclically):
JavaScript
var sgart = sgart || {};
sgart.assignValuesCyclically = function(id, seconds, values, isClass){
if (typeof values!=='object') values = ['#00f','#0f0','#f00']; // default
if (typeof isClass!=='boolean') isClass = false; // default color
var maxIndex = values.length;
var index = 0;
function setValue(){
console.log(values[index]);
if(isClass)
document.getElementById(id).className = values[index];
else
document.getElementById(id).style.backgroundColor = values[index];
index++;
if(index>=maxIndex) index=0;
}
setValue();
setInterval(setValue, seconds*1000);
};
sgart.it - test 1
sgart.it - test 2
sgart.it - test 3
sgart.it - test 4
JavaScript
sgart.assignValuesCyclically('test1', .2, ['#442200','#552B00','#663300','#773C00','#884400','#994D00','#AA5500','#BB5E00','#CC6600','#DD6F00','#EE7700']);
JavaScript
sgart.assignValuesCyclically('test2', 2);
JavaScript
sgart.assignValuesCyclically('test3', 1.5, ['class-1 class-2','class-a','my-class'],true);
L'ultimo esempio test 4 utilizza una diversa versione dello script che è la seguente (sgart.assignValuesCyclicallyEnhanced):
JavaScript
sgart.assignValuesCyclicallyEnhanced = function(id, values, isClass){
if (typeof values!=='object') values = [{v:'#00f', s:1},{v:'#0f0',s:2},{v:'#f00',s:3}];
if (typeof isClass!=='boolean') isClass = false;
var maxIndex = values.length;
var index = 0;
function setValue(){
var v = values[index];
console.log(v);
if(isClass)
document.getElementById(id).className = v.v;
else
document.getElementById(id).style.backgroundColor = v.v;
index++;
if(index>=maxIndex) index=0;
setTimeout(setValue, v.s*1000);
}
setValue();
};
JavaScript
sgart.assignValuesCyclicallyEnhanced('test4', [{v:'#6633FF',s:1},{v:'#773C88',s:3},{v:'#884400',s:1}]);
Tramite il test typeof values!=='undefined' è possibile gestire funzioni con numero di parametri variabili e impostare i valori di default nel caso non fossero passati
Con le opportune modifiche è possibile gestire anche il cambio del source di un immagine o l'immagine di sfondo di un elemento HTML:
JavaScript
document.getElementsByName(id)[0].src = v.v; //document.getElementsByName(id)[0].src = 'myimg.png';
document.body.style.backgroundImage = v.v; //document.body.style.backgroundImage = "url('myimg.png')";
Usando le classi (ultimo parametro a true) si possono creare semplici animazioni, con tempo di transizione variabile, basate sui CSS
Il codice HTML usato per l'esempio è il seguente:
HTML
<!-- classi usate nell'esempio test 3 -->
<style>
.class-1 { font-size: 18pt; }
.class-2 { color: green; }
.class-a { background-color: yellow; text-align: center; font-weight: bold; padding: 10px; }
.my-class { border: 1px solid #f00; text-align: right; }
</style>
<hr/>
<div id="test1">sgart.it - test 1</div>
<hr/>
<div id="test2">sgart.it - test 2</div>
<hr/>
<div id="test3">sgart.it - test 3</div>
<hr/>
<div id="test4">sgart.it - test 4</div>
<hr/>
Le funzioni usano solo JavaScript e non richiedono librerie esterne