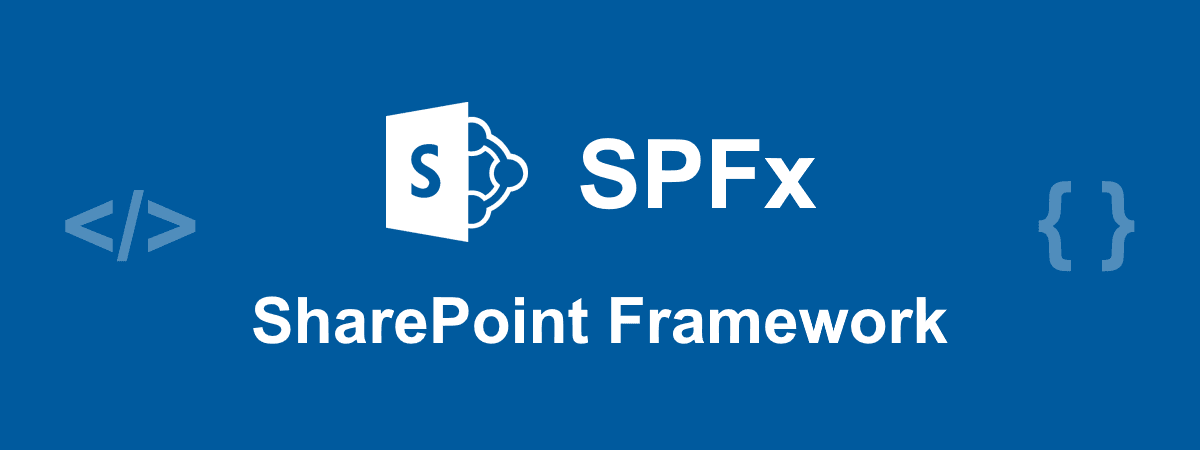
Chiamare una Rest API di SharePoint Online da SPFx
In una webpart SPFx è possibile richiamare una Rest API di SharePoint Online come questa
tramite l'oggetto spHttpClient recuperato dal context
che ritorna un JSON simile a questo
nell'header vanno indicate le intestazioni (vedi oggetto _httpOptionsGet)
dove con odata=nometadata si richiede una risposta dell'API compatta senza metadati aggiuntivi.
Si può gestire la risposta (oggetto responseJson) controllando anche eventuali errori:
viene stampato in console un oggetto simile a questo
Il path /_api/ va aggiunto dopo la site collection.
Dopo va aggiunto l'oggetto che si vuole interrogare (/lists, /folders, /webinfows) e poi l'eventuale metodo (/GetByTitle, /GetList o (guid'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx')) con l'eventuale parametro (Test Configurable View) che identifica la specifica risorsa.
In questo caso voglio leggere gli items della lista quindi aggiungo il path /items.
Questa sintassi permette di identificare in modo univoco la risorsa a cui si vuole accedere.
Specificando dei parametri in querystring, dopo il punto di domanda, è possibile:
Per un dettaglio completo sulla sintassi dell'API vedi Get to know the SharePoint REST service.
Text: Esempio API Rest
https://TenantName.sharepoint.com/sites/SiteCollectionName/_api/web/lists/GetByTitle('Test Configurable View')/items?$select=Id,Title,Description,DataPub,Author/Title,Image1,PageUrl,OpenInNewWindow,InEvidence&$expand=Author&$orderby=Title&$top=6
TypeScript
import { WebPartContext } from "@microsoft/sp-webpart-base";
import { SPHttpClient, SPHttpClientResponse, ISPHttpClientOptions } from '@microsoft/sp-http';
const _httpOptionsGet: ISPHttpClientOptions = {
headers: {
'odata-version': '3.0',
'accept': 'application/json;odata=nometadata',
}
};
const url = "/_api/web/lists/GetByTitle('Test Configurable View')/items"
+ "?"
+ "$select=Id,Title,Description,DataPub,Author/Title,Image1,PageUrl,OpenInNewWindow,InEvidence"
+ "&$expand=Author"
+ "&$orderby=Title"
+ "&$top=6";
const response: SPHttpClientResponse = await this.context.spHttpClient.get(
url,
SPHttpClient.configurations.v1,
_httpOptionsGet
);
const responseJson = await response.json();
JSON: Response
{
"odata.nextLink": "https://TenantName.sharepoint.com/_api/web/lists/GetByTitle(%27Test%20Configurable%20View%27)/items?%24skiptoken=Paged%3dTRUE%26p_ID%3d2&%24select=Id%2cTitle%2cDescription%2cDataPub%2cAuthor%2fTitle%2cImage1%2cPageUrl%2cOpenInNewWindow%2cInEvidence&%24expand=Author&%24top=2",
"value": [
{
"Author": {
"Title": "Alberto "
},
"Id": 1,
"ID": 1,
"Title": "Sgart.it",
"Description": "sito pubblico",
"Image1": "{\"fileName\":\"sgart-polygon-03.jpg\",\"serverRelativeUrl\":\"/SiteAssets/Lists/0cd354e9-503e-4e02-8c0d-fa8f55d6f073/sgart-polygon-03.jpg\",\"id\":\"ccbe391b-4a0b-4e3f-82d7-1ce82e382810\",\"serverUrl\":\"https://TenantName.sharepoint.com\",\"thumbnailRenderer\":{\"spItemUrl\":\"https://sgart.sharepoint.com:443/_api/v2.1/drives/b!vw5MPoSoZkahFypdPMrOSha-aiBMjoN...WmuefMgb/items/01TUXGX...5AXDQKAQ\",\"fileVersion\":2,\"sponsorToken\":\"L0xpc3...YWdlMXwx\"},\"type\":\"thumbnail\",\"fieldName\":\"Image1\",\"fieldId\":\"f48d6288-...3294830e12\"}",
"PageUrl": {
"Description": "Sgart.it",
"Url": "https://www.sgart.it"
},
"OpenInNewWindow": true,
"DataPub": "2000-12-31T23:00:00Z",
"InEvidence": null
},
{
"Author": {
"Title": "Alberto"
},
"Id": 2,
"ID": 2,
"Title": "SharePoint",
"Description": "sito test",
"Image1": null,
"PageUrl": {
"Description": "https://TenantName.sharepoint.com/",
"Url": "https://TenantName.sharepoint.com/"
},
"OpenInNewWindow": false,
"DataPub": "2019-02-13T23:00:00Z",
"InEvidence": null
}
]
}
Text: Headers
odata-version: 3.0
accept: application/json;odata=nometadata
Si può gestire la risposta (oggetto responseJson) controllando anche eventuali errori:
TypeScript
if (responseJson['odata.error'] !== undefined) {
console.error(ERROR_PREFIX + result.responseJson['odata.error']['message']['value']);
} else {
const spItems = result.responseJson.value;
if (spItems === undefined) {
console.error(`${ERROR_PREFIX}Response 'value' undefined, please check parameters and generated URL`);
} else {
const items = spItems.map((item: any): IItem => {
const title = getString(item, fields.title);
return {
id: item.Id,
title: isNullOrWhiteSpace(title) || title === '-' ? '' : title,
description: getString(item, fields.description),
date: getDate(item, fields.date),
user: getString(item, fields.user),
image: getString(item, fields.image),
url: getString(item, fields.url),
targetBlank: getBoolean(item, fields.targetBlank),
inEvidence: getBoolean(item, fields.inEvidence)
};
});
console.log(items);
}
}
JSON
[
{
"id": 1,
"title": "Sgart.it",
"description": "sito pubblico",
"date": "01/01/2001",
"user": "Alberto",
"image": "{"fileName":"sgart-polygon-03.jpg","serverRelativeUrl":"/SiteAssets/Lists/0cd354e9-503e-4e02-8c0d-fa8f55d6f073/sgart-polygon-03.jpg","id":"ccbe391b-4a0b-4e3f-82d7-1ce82e382810","serverUrl":"https://sgart.sharepoint.com","thumbnailRenderer":{"spItemUrl":"https://TenantName.sharepoint.com:443/_api/v2.1/drives/b!vw5MPoSo...PMrOSha-aiBMjo...uBCSZwDWmuefMgb/items/01TUXGX4A3..Y45AXDQKAQ","fileVersion":2,"sponsorToken":"L0xpc3RzL1Rlc3Rjb25...V3fEltYWdlMXwx"},"type":"thumbnail","fieldName":"Image1","fieldId":"f48d6288-2426-...0d-0b3294830e12"}",
"url": "https://www.sgart.it",
"targetBlank": true,
"inEvidence": null
},
{
"id": 2,
"title": "SharePoint",
"description": "sito test",
"date": "14/02/2019",
"user": "Alberto",
"image": null,
"url": "https://TenantName.sharepoint.com/",
"targetBlank": false,
"inEvidence": null
}
]
Utility
L/esempio utilizza le seguenti funzioni comuniTypeScript
let _locale: string = 'it-IT';
const _localeDateOptions: Intl.DateTimeFormatOptions = { year: 'numeric', month: '2-digit', day: '2-digit' };
export const isNullOrWhiteSpace = (str: string): boolean => {
if (undefined === str || null === str) return true;
if ((/^\s*$/g).test(str)) return true;
return false;
};
const getFieldParams = (item: object, name: string): IFieldParams => {
if (undefined === name || null === name || name.length === 0) return null;
return {
name,
value: item[name]
};
};
const getString = (item: object, name: string): string | null => {
const p = getFieldParams(item, name);
if (null === p || undefined === p.value || null === p.value) return null;
return p.value;
};
const getDate = (item: object, name: string): string | null => {
const p = getFieldParams(item, name);
if (null === p || undefined === p.value || null === p.value) return null;
return date.toLocaleString(_locale, _localeDateOptions);
};
const getBoolean = (item: object, name: string): boolean => {
const p = getFieldParams(item, name);
if (null === p || undefined === p.value || null === p.value) return null;
return p.value === true || p.value === 'true';
};
Rest API
L'API di SharePoint Online per accedere ai dati delle liste ha il seguente formato:Text: Esempio API Rest
https://TenantName.sharepoint.com/sites/SiteCollectionName/_api/web/lists/GetByTitle('Test Configurable View')/items?$select=Id,Title,Description,DataPub,Author/Title,Image1,PageUrl,OpenInNewWindow,InEvidence&$expand=Author&$orderby=Title desc&$top=6
Dopo va aggiunto l'oggetto che si vuole interrogare (/lists, /folders, /webinfows) e poi l'eventuale metodo (/GetByTitle, /GetList o (guid'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx')) con l'eventuale parametro (Test Configurable View) che identifica la specifica risorsa.
In questo caso voglio leggere gli items della lista quindi aggiungo il path /items.
Questa sintassi permette di identificare in modo univoco la risorsa a cui si vuole accedere.
Specificando dei parametri in querystring, dopo il punto di domanda, è possibile:
- scegliere di far ritornare solo alcuni campi $select
- filtrare gli oggetti con il parametro $filters
- espandere i campi complessi come Lookup o User con il parametro $expand
- ordinare il risultato tramite il parametro $orderby
- limitare il risultato tramite il parametro $top
Per un dettaglio completo sulla sintassi dell'API vedi Get to know the SharePoint REST service.