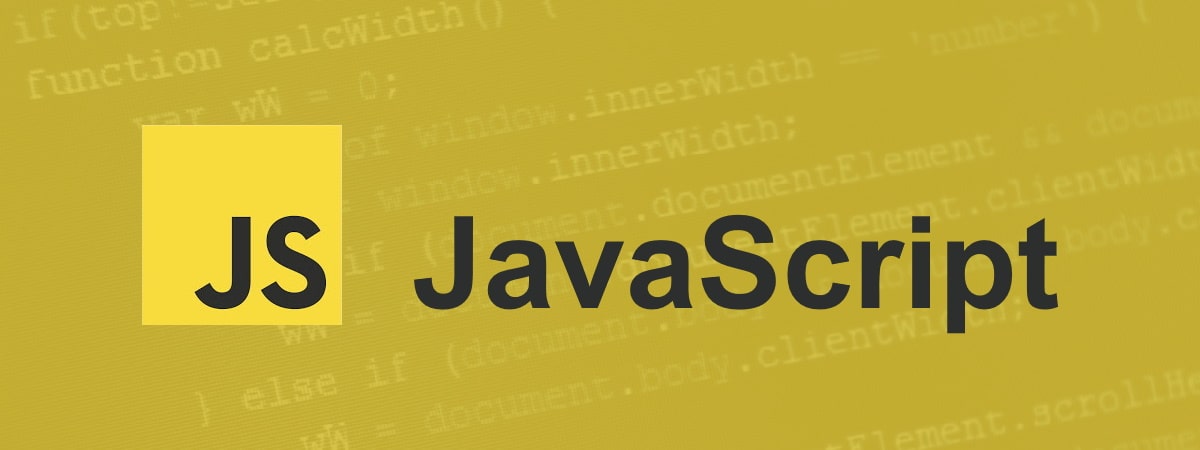
Chiamata Ajax in JavaScript senza framework (XMLHttpRequest) - Vanilla JS
Con i browser moderni è possibile eseguire delle chiamate AJAX in JavaScript senza l'utilizzo di librerie esterne (Vanilla JS).
La chiamata può essere fatta tramite l'oggetto XMLHttpRequest presente in tutti i browser.
la funzione può essere usata in questo modo:
l'uso della funzione sarà:
per usarla devo avere una tag input di tipo file in pagina
e posso eseguire l'upload con questo codice
La chiamata può essere fatta tramite l'oggetto XMLHttpRequest presente in tutti i browser.
GET Json
La funzione sgart.jsonGet è un esempio di chiamata AJAX di tipo GET per ottenere in risposta un oggetto JSON:JavaScript
var sgart = sgart || {};
/*
* Sgart.it - chiamata ajax in GET
* url: url del servizio da chiamare con eventuali parametri in query string
* fnCallbackOk: funzione richiamata in caso di successo
* fnCallbackError: funzione richiamata in caso di errore
*/
sgart.jsonGet = function (url, fnCallbackOk, fnCallbackError) {
// creo l'oggetto per la chiamata
var xHttp = new XMLHttpRequest();
// gestisco la callback di risposta
xHttp.onreadystatechange = function () {
if (this.readyState === 4) {
if (this.status === 200) {
if (typeof fnCallbackOk === "function") {
// se ho un 200 tutto ok,
// chiamo la funzione passata di callback passandogli l'oggetto json
fnCallbackOk(JSON.parse(this.responseText));
} else {
// se non ho passato una funzione stampo un warning in console;
console.log("warning: reponse ok but no calback function", url);
}
} else {
// se sono qui c'è stato un errore, lo scrivo sempre in console
console.log("Error", url, this.status, this.responseText);
if (typeof fnCallbackError === "function") {
// se esiste chiamo la callback di errore
fnCallbackError(this.status, this.responseText);
}
}
}
};
// inposto la chiamata in GET asincrona (true)
xHttp.open("GET", url, true);
// imposto il content type per le chiamate json
xHttp .setRequestHeader("Content-type", "application/json");
// header necessario per le chiamate alla API di SharePoint
xHttp .setRequestHeader("Accept", "application/json; odata=verbose");
// eseguo la chiamata
xHttp.send();
};
JavaScript
sgart.jsonGet(
'./sgart-template-examples.json',
function (responseJson) {
console.log('ok', responseJson)
},
function (status, responseText) {
console.log('error', status, responseText)
}
);
POST Json
Allo stesso modo si possono gestire le chiamate in POST tramite la funzione sgart.jsonPost:JavaScript
/*
* Sgart.it - chiamata ajax in POST
* url: url del servizio da chiamare
* jsonObject: oggetto javascript
* fnCallbackOk: funzione richiamata in caso di successo
* fnCallbackError: funzione richiamata in caso di errore
*/
sgart.jsonPost = function (url, jsonObject, fnCallbackOk, fnCallbackError) {
var xHttp = new XMLHttpRequest();
xHttp.onreadystatechange = function () {
if (this.readyState === 4) {
if (this.status === 200) {
if (typeof fnCallbackOk === "function") {
fnCallbackOk(JSON.parse(this.responseText));
} else {
console.log("warning: reponse ok but no calback function", url);
}
} else {
console.log("Error", url, this.status, this.responseText);
if (typeof fnCallbackError === "function") {
fnCallbackError(this.status, this.responseText);
}
}
}
};
// imposto la chiamata in POST asincrona
xHttp.open("POST", url, true);
xHttp.setRequestHeader("Content-type", "application/json");
// header necessario per le chiamate alla API di SharePoint
xHttp .setRequestHeader("Accept", "application/json; odata=verbose");
// serializzo l'oggetto json in stringa
var data = JSON.stringify(jsonObject);
// invio la chiamata in post con la stringa che rappresenta il json
xHttp.send(data);
};
JavaScript
// oggetto da passare in post
var data = {
id: 1,
title: 'prova post'
};
sgart.jsonPost('./api/save', data, function (responseJson) {
console.log('ok', responseJson)
}
/* se non serve posso evitare la callback di errore
l'eventuale errore verrà comunque scritto in console */
);
Post Files
Se invece devo fare l'upload dei file, posso usare questa funzione sgart.formDataPostJavaScript
/*
* Sgart.it - chiamata ajax in POST
* url: url del servizio da chiamare
* formData: oggetto javascript (es. new FormData();)
* fnCallbackOk: funzione richiamata in caso di successo
* fnCallbackError: funzione richiamata in caso di errore
*/
sgart.formDataPost = function (url, formData, fnCallbackOk, fnCallbackError) {
var xHttp = new XMLHttpRequest();
xHttp.onreadystatechange = function () {
if (this.readyState === 4) {
if (this.status === 200) {
if (typeof fnCallbackOk === "function") {
fnCallbackOk(this.responseText);
} else {
console.log("warning: reponse ok but no calback function", url);
}
} else {
console.log("Error", url, this.status, this.responseText);
if (typeof fnCallbackError === "function") {
fnCallbackError(this.status, this.responseText);
}
}
}
};
// imposto la chiamata in POST asincrona
xHttp.open("POST", url, true);
var data = formData;
// invio la chiamata in post con il FormData
xHttp.send(data);
};
HTML
<input type="file" id="sgart-file" multiple>
JavaScript
// recupero il riferimento al campo input di tipo file
var files = document.getElementById("sgart-file").files;
// url su cui fare l'upload
var url ="https://localhost:44300/api/v1/forum/";
// preparo i file da inviare
var formData = new FormData();
for(var i=0; i<files.length; i++){
console.log("File", files[i]);
formData.append("files", files[i]);
}
// upload dei files
sgart.formDataPost(url, formData, function(response) {
console.log("Response", response);
});