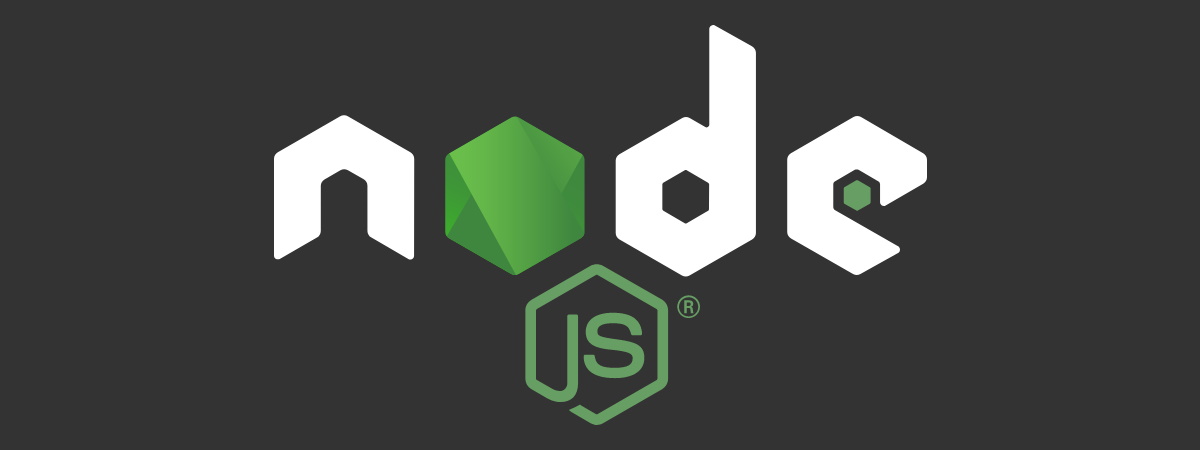
Cominciare ad usare Node.js
Node.js è un piattaforma per eseguire codice JavaScript non bloccante (asincrono) lato server (non nel browser) ed è basato sul motore JavaScript V8 di Chrome.
Per installarlo vai sul sito di Node.js e installalo per la tua piattaforma in quanto supporta Windows, Linux e macOS (as esempio installalo in c:\nodejs).
Si tratta di un eseguibile di circa 10MB. Con Node.js viene installato anche npm (Node Package Manager in c:\nodejs\node_modules\npm). Npm è una utility che permette di gestire e installare pacchetti (componenti aggiuntivi per Node.js).
Una volta installato apri la console Node.js command prompt che imposta già le variabili di ambiente (su linux o mac una qualsiasi shell), poi esegui il comando node. In questo modo entri nella modalità interattiva in cui puoi inserire qualsiasi comando JavaScript:
L'esempio da come risultato 3.
Questo è un esempio di un semplice web server che ritorna file statici presenti nella cartella corrente:
esegui il webserver con:
poi apri un browser e inserisci http://127.0.0.1:4000/webserver1.js
verrà visualizzato il file.
Qualunque file presente nella cartella viene ritornato.
Per utilizzare Microsoft SQL Server, serve il modulo node-mssql, quindi digita:
per eseguirlo:
otterrai come risultato la stampa del contenuto della query:
Node.js è supportato anche da Microsoft su Azure.
Per installarlo vai sul sito di Node.js e installalo per la tua piattaforma in quanto supporta Windows, Linux e macOS (as esempio installalo in c:\nodejs).
Si tratta di un eseguibile di circa 10MB. Con Node.js viene installato anche npm (Node Package Manager in c:\nodejs\node_modules\npm). Npm è una utility che permette di gestire e installare pacchetti (componenti aggiuntivi per Node.js).
L'ho provato con successo sia su Windows7 e 8, Linux Ubuntu, Mac OS e Rasberry PI 2B. Il codice che ho testato ha funzionato su tutte le piattaforme semplicemente copiando i files.
Una volta installato apri la console Node.js command prompt che imposta già le variabili di ambiente (su linux o mac una qualsiasi shell), poi esegui il comando node. In questo modo entri nella modalità interattiva in cui puoi inserire qualsiasi comando JavaScript:
Text
var a=1;
var b=Math.abs(-2);
console.log(a+b);
Se esegui un prompt dei comandi diverso (non quello di node) digita c:\nodejs\nodevars.bat per settare le variabili di ambiente
Ovviamente la modalità interattiva non è quella interessante ma prima di vedere altri esempi e meglio se scarichi nodemon. Si tratta di un tool per eseguire Node.js in modalità debug, che si riavvia ad ogni cambiamento dei file di progetto:DOS / Batch file
npm install nodemon -g
l'opzione -g installa i pacchetti globalmente in C:\Users\nomeUtente\AppData\Roaming\npm\node_modules
Questo è un esempio di un semplice web server che ritorna file statici presenti nella cartella corrente:
JavaScript: webserver1.js
//richiedo i moduli necessati (tutti standard già presenti in node)
var http = require('http');
var fs = require('fs');
var path = require('path');
//setto la porta in cui sarà in ascolto il webserver
//se non è presente nella variabile di ambiente PORT
var port=process.env.PORT || '4000';
//creo il web server
http.createServer(function (request, response) {
console.log('request starting ' + request.url + '...'); //visualizzo nella console
var filePath = '.' + request.url;
if (filePath == './')
filePath = './index.html'; //di default cerco la pagina index.html nella root
var extname = path.extname(filePath);
var contentType = 'text/html';
//setto il content type da ritornare in base all`estensione
switch (extname) {
case '.js':
contentType = 'text/javascript';
break;
case '.css':
contentType = 'text/css';
break;
case '.json':
contentType = 'application/json';
break;
case '.png':
contentType = 'image/png';
break;
case '.jpg':
contentType = 'image/jpg';
break;
case '.wav':
contentType = 'audio/wav';
break;
}
//leggo il file
fs.readFile(filePath
, function(error, content) { //funzione di calback per gestire la lettura del file, tutto asincrono
if (error) {
//gestione errori
if(error.code == 'ENOENT'){
//se non trovo il file richiesto visualizzo la pagina 404.html
fs.readFile('./404.html', function(error, content) {
response.writeHead(200, { 'Content-Type': contentType });
response.end(content, 'utf-8');
});
}
else {
response.writeHead(500);
response.end('Sorry, error: '+error.code+' ..\n');
response.end();
}
}
else {
// se non ho errori ritorno il contenuto del file
response.writeHead(200, { 'Content-Type': contentType });
response.end(content, 'utf-8');
}
});
}).listen(port); //imposto il server per ascoltare sulla porta indicata
console.log('Server running at http://127.0.0.1:'+port+'/');
DOS / Batch file
nodemon werbserver1.js
Qualunque file presente nella cartella viene ritornato.
avendolo eseguito con il comando nodemon qualunque cambiamento al file webserver1.js provocherà il riavvio dell'applicazione con il nuovo contenuto. Come editor multi piattaforma puoi usare Visual Studio code
Puoi usarlo anche per accedere a Microsoft SQL Server, MySQL o altri DB.Per utilizzare Microsoft SQL Server, serve il modulo node-mssql, quindi digita:
DOS / Batch file
c:
cd \nodejs
md testsql
cd testsql
npm i mssql
questa volta non ho usato il parametro -g del comando npm e quindi il package viene scaricato nella directory c:\nodejs\node_modules\mssql
poi crea il file app.js nella cartella testsql:JavaScript
var sql = require('mssql'); // assicurati di aver abilitato il protocollo tcp di sql su una porta specifica
var config = {
user: 'nodejs',
password: 'nodejspassword',
server: 'localhost',
database: 'AdventureWorks2012',
options: {
//InstanceName: 'SQLEXPRESS', //questa proprietà non sembra necessaria
port: 1433, // va sempre indicata la porta (non funziona con le porte dinamiche)
encrypt: false // Use this if you`re on Windows Azure
}
};
var connection = new sql.Connection(config, function (err) {
console.log('connection: '+ JSON.stringify(connection)); //visualizzo in console parametri di connessione
var query='SELECT StateProvinceID, Name FROM [Person].[StateProvince] WHERE CountryRegionCode=@code ORDER BY Name';
var ps = new sql.PreparedStatement(connection);
ps.input('code', sql.VarChar(3)); //definisco un parametro da passare alla query
ps.prepare(query, function (err) { //preparo la query
if (err) throw err; //verifico che non ci siano errori
//eseguo la query
ps.execute({
code: 'CA' //passo il valore da assegnare alla variabile @code
}, function (err, recordset) { //creo una calback per gestire la risposta (tutto asincrono non bloccante)
if (err) throw err;
ps.unprepare(function (err) { //distruggo l'oggetto prepare altrimenti la connessione non viene liberata
if (err) throw err;
});
// stampo nella console i dati ritornati
console.log(recordset);
});
});
});
DOS / Batch file
nodemon app.js
Text
connection: {"config":{"user":"nodejs","password":"nodejspassword","server":"localhost","database":"AdventureWorks2012","options":{"port":1433,"encrypt":false,"database":"AdventureWorks2012","connectTimeout":15000,"requestTimeout":15000,"tdsVersion":"7_4","rowCollectionOnDone":false,"rowCollectionOnRequestCompletion":false
,"useColumnNames":false,"appName":"node-mssql","textsize":"2147483647","cancelTimeout":5000,"packetSize":4096,"isolationLevel":2,"cryptoCredentialsDetails":{},"
useUTC":true,"connectionIsolationLevel":2,"readOnlyIntent":false,"enableAnsiNullDefault":true},"driver":"tedious","port":1433,"stream":false},"driver":{},"connecting":false,"pool":{},"connected":true,"_events":{}}
[ { StateProvinceID: 1, Name: 'Alberta' },
{ StateProvinceID: 7, Name: 'British Columbia' },
{ StateProvinceID: 41, Name: 'Brunswick' },
{ StateProvinceID: 29, Name: 'Labrador' },
{ StateProvinceID: 31, Name: 'Manitoba' },
{ StateProvinceID: 45, Name: 'Newfoundland' },
{ StateProvinceID: 51, Name: 'Northwest Territories' },
{ StateProvinceID: 49, Name: 'Nova Scotia' },
{ StateProvinceID: 57, Name: 'Ontario' },
{ StateProvinceID: 60, Name: 'Prince Edward Island' },
{ StateProvinceID: 63, Name: 'Quebec' },
{ StateProvinceID: 69, Name: 'Saskatchewan' },
{ StateProvinceID: 83, Name: 'Yukon Territory' } ]
Questo esempio l'ho anche copiato su Raspberry PI 2 in cui avevo già installato Node.js.
Poi ho installato il package mssql con npm e cambiato la stringa di connessione al DB per puntare all'IP di un SQL Server Express che ho su windows.
L'ultimo passo è stato aprire la porta TCP 1433 sul firewall windows e tutto ha funzionato correttamente.
Poi ho installato il package mssql con npm e cambiato la stringa di connessione al DB per puntare all'IP di un SQL Server Express che ho su windows.
L'ultimo passo è stato aprire la porta TCP 1433 sul firewall windows e tutto ha funzionato correttamente.
Gli esempi sono basati sulla versione v0.12.5 di Node.js (node -v).
Prossimamente pubblicherò altri esempi con Node.js ed express un framework per gestire applicazioni web.Node.js è supportato anche da Microsoft su Azure.