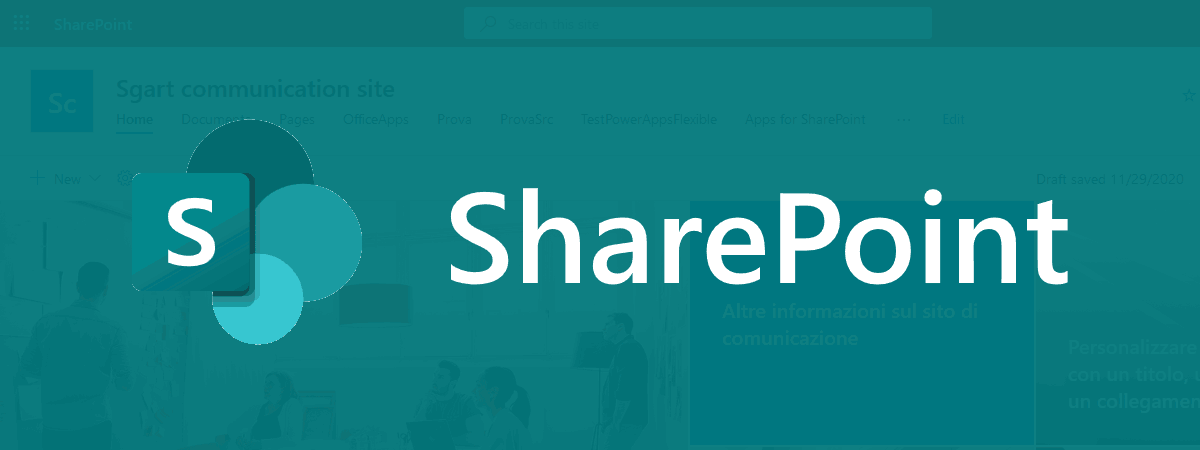
Generatore di istruzioni Add-PnPField per site column SharePoint
Questo script PowerShell permette di leggere, da uno SharePoint Online, le colonne (Filed) a livello di site collection, appartenenti ad uno specifico gruppo, e generare le istruzioni PnP per ricreare le stesse su un altra site collection
genera un output simile a questo
I comandi generati possono essere salvati su un file .ps1 con questo comando:
Vedi anche Generatore di istruzioni Add-PnPContentType per site content type SharePoint
PowerShell: sgart-generate-fields.ps1
# Genera istruzioni PnP per la creazione di colonne di sito in una diversa site collection - Sgart.it
# https://www.sgart.it/IT/informatica/generatore-di-istruzioni-add-pnpfield-per-site-column-sharepoint/post
# Moduli necessari: Install-Module -Name PnP.PowerShell
# es.: .\sgart-generate-fields.ps1 "https://tenantXX.sharepoint.com" "nome gruppo" > .\sgart-generate-fields-dest.ps1
param (
[Parameter(Mandatory=$true)]
[string]$siteCollectionUrlSource,
[Parameter(Mandatory=$true)]
[string]$fieldsGroupName
)
#$siteCollectionUrlSource = "https://tenantXX.sharepoint.com"
#$fieldsGroupName = "Sgart test export"
Write-Host "siteCollectionUrlSource: $siteCollectionUrlSource"
Write-Host "fieldsGroupName: $fieldsGroupName"
Connect-PnPOnline -Url $siteCollectionUrlSource -Interactive
function EscapeString {
param (
[string]$str
)
$str.Replace("`"", "```"").Replace("`r", "``r").Replace("`n", "``n").Replace("`t", "``t");
}
function BuildPropertyString {
param (
[string]$variableName,
[string]$propertyName,
[string]$propertyValue
)
if ($false -eq [string]::IsNullOrWhiteSpace($propertyValue)){
"$($variableName).$($propertyName) = `"$(EscapeString($propertyValue))`";`n"
} else {
""
}
}
function BuildPropertyBool {
param (
[string]$variableName,
[string]$propertyName,
[string]$propertyValue
)
if ($true -eq $propertyValue){
"$($variableName).$($propertyName) = `$true;`n"
} else {
""
}
}
function BuildPropertyNumber {
param (
[string]$variableName,
[string]$propertyName,
[string]$propertyValue
)
if ($true -eq $propertyValue){
"$($variableName).$($propertyName) = $propertyValue;`n"
} else {
""
}
}
function BuildPropertyChoices {
param (
[string]$variableName,
[string[]]$propertyValue
)
if ($propertyValue.Length -gt 0) {
$s = "$($variableName).Choices.Clear();`n"
$propertyValue | ForEach-Object {
$s += "$($variableName).Choices.Add(`"$(EscapeString($_))`");`n"
}
$s
} else {
""
}
}
function BuilAddfiledXml {
param (
[string]$variableName,
[string]$propertyValue
)
[xml]$x= $propertyValue;
$x.Field.Version = "";
$x.Field.Group = "`$fieldsGroupName";
if($null -ne $x.Field.CustomFormatter) {
$x.Field.CustomFormatter = $x.Field.CustomFormatter.Replace('$', '`$')
}
$str = $x.OuterXml.Replace("Version=`"`"", "").Replace("`"", "```"")
"$variableName = Add-PnPFieldFromXml -FieldXml `"$str`";"
}
Write-Output "#==========================================================================================="
Write-Output "# Sgart.it - https://www.sgart.it/IT/informatica/generatore-di-istruzioni-add-pnpfield-per-site-column-sharepoint/post"
Write-Output "# Generato il $(Get-Date -format "yyyy-MM-dd HH:mm:ss")"
Write-Output "# "
Write-Output "# ATTENZIONE: modifica le variabili per la connessione al nuovo tenant/site collection !!!!!"
Write-Output "#==========================================================================================="
Write-Output "`$siteCollectionUrlDest = `"https://<tenant-di-destinazione>.sharepoint.com/<eventuale-site-collection-url>`";"
Write-Output "`$fieldsGroupName = `"$($fieldsGroupName)`";"
Write-Output " "
Write-Output "Write-Output `"Url: `$siteCollectionUrlDest, Group: `$fieldsGroupName`";"
Write-Output "Connect-PnPOnline -Url `$siteCollectionUrlDest -Interactive;"
Write-Output " "
# esporta tutti i campi e genera le istruzioni di creazione
Write-Output "#==========================================================================================="
Write-Output "# istruzioni di creazione dei campi di site collection"
Write-Output "#==========================================================================================="
Write-Output " "
$fields = Get-PnPField | Where-Object {$_.Group -eq $fieldsGroupName}
$i=1
$m = $fields.Count
$fields | ForEach-Object {
$fld = $_
$fldVarName = "`$fldAdd$($i)"
$internalName = $fld.InternalName
$type = $fld.TypeAsString
$msg = "$i / $m) $fldVarName - $internalName - $type ..."
Write-Host $msg
Write-Output "Write-Output `"$msg`";"
$fldCmd = "";
$fldCmd = BuilAddfiledXml $fldVarName $fld.SchemaXml
Write-Output $fldCmd
$fldCmdOpt ="";
$fldCmdOpt += BuildPropertyString $fldVarName "Description" $fld.Description
if($fldCmdOpt.Length -gt 0){
$fldCmdOpt += "$($fldVarName).Update();"
Write-Output $fldCmdOpt
}
Write-Output ""
$i++
}
PowerShell: Script generato
#===========================================================================================
# Sgart.it - https://www.sgart.it/IT/informatica/generatore-di-istruzioni-add-pnpfield-per-site-column-sharepoint/post
# Generato il 2021-11-03 00:39:19
#
# ATTENZIONE: modifica le variabili per la connessione al nuovo tenant/site collection !!!!!
#===========================================================================================
$siteCollectionUrlDest = "https://<tenant-di-destinazione>.sharepoint.com/<eventuale-site-collection-url>";
$fieldsGroupName = "Sgart test export";
Write-Output "Url: $siteCollectionUrlDest, Group: $fieldsGroupName";
Connect-PnPOnline -Url $siteCollectionUrlDest -Interactive;
#===========================================================================================
# istruzioni di creazione dei campi di site collection
#===========================================================================================
Write-Output "1 / 4) $fldAdd1 - SgartFldNumber - Number ...";
$fldAdd1 = Add-PnPFieldFromXml -FieldXml "<Field Type=`"Number`" DisplayName=`"Fld Number`" Required=`"TRUE`" EnforceUniqueValues=`"FALSE`" Indexed=`"FALSE`" Percentage=`"TRUE`" Group=`"$fieldsGroupName`" ID=`"{ea30d241-1cfd-47bf-a1ee-6556b42f67bf}`" SourceID=`"{206abe16-8e4c-4883-8161-bbc06ecf94be}`" StaticName=`"SgartFldNumber`" Name=`"SgartFldNumber`" CustomFormatter=`"`"><Default>.05</Default></Field>";
Write-Output "2 / 4) $fldAdd2 - SgartFldText - Text ...";
$fldAdd2 = Add-PnPFieldFromXml -FieldXml "<Field Type=`"Text`" DisplayName=`"Fld Text`" Required=`"TRUE`" EnforceUniqueValues=`"FALSE`" Indexed=`"FALSE`" MaxLength=`"255`" Group=`"$fieldsGroupName`" ID=`"{ae84c8f2-1377-47ff-92cb-9c867410b04c}`" SourceID=`"{206abe16-8e4c-4883-8161-bbc06ecf94be}`" StaticName=`"SgartFldText`" Name=`"SgartFldText`" CustomFormatter=`"{
 "`$schema": "https://developer.microsoft.com/json-schemas/sp/v2/column-formatting.schema.json",
 "elmType": "div",
 "txtContent": "@currentField"
}`" Description=`"Descrizione con "virgolette doppie".`"><Default>testo prova 1</Default></Field>";
$fldAdd2.Description = "Descrizione con `"virgolette doppie`".";
$fldAdd2.Update();
Write-Output "3 / 4) $fldAdd3 - SgartFldTextMulti - Note ...";
$fldAdd3 = Add-PnPFieldFromXml -FieldXml "<Field Type=`"Note`" DisplayName=`"Fld Text Multi`" Required=`"FALSE`" EnforceUniqueValues=`"FALSE`" Indexed=`"FALSE`" NumLines=`"10`" RichText=`"TRUE`" RichTextMode=`"FullHtml`" IsolateStyles=`"TRUE`" Sortable=`"FALSE`" Group=`"$fieldsGroupName`" ID=`"{91d20f14-e431-46ae-9e9e-db14a0be12ba}`" SourceID=`"{206abe16-8e4c-4883-8161-bbc06ecf94be}`" StaticName=`"SgartFldTextMulti`" Name=`"SgartFldTextMulti`" CustomFormatter=`"`" RestrictedMode=`"TRUE`" AppendOnly=`"FALSE`" UnlimitedLengthInDocumentLibrary=`"FALSE`"></Field>";
Write-Output "4 / 4) $fldAdd4 - SgartFldChoice - Choice ...";
$fldAdd4 = Add-PnPFieldFromXml -FieldXml "<Field Type=`"Choice`" DisplayName=`"SgartFldChoice`" Required=`"FALSE`" EnforceUniqueValues=`"FALSE`" Indexed=`"FALSE`" Format=`"Dropdown`" FillInChoice=`"FALSE`" Group=`"$fieldsGroupName`" ID=`"{e085bd70-b147-4faa-9273-8f33038eeed9}`" SourceID=`"{206abe16-8e4c-4883-8161-bbc06ecf94be}`" StaticName=`"SgartFldChoice`" Name=`"SgartFldChoice`" ><Default>Scelta 2</Default><CHOICES><CHOICE>Scelta 1</CHOICE><CHOICE>Scelta 2</CHOICE><CHOICE>Scelta 3</CHOICE></CHOICES></Field>";
Non sono gestiti tutti i tipi di campi, come ad esempio le lockup.
Testato solo con SharePoint Online.
I comandi generati possono essere salvati su un file .ps1 con questo comando:
PowerShell
.\sgart-generate-fields.ps1 "https://tenantXX.sharepoint.com/siteCollection" "nome gruppo" > ".\sgart-generate-fields-dest_$(Get-Date -format "yyyy-MM-dd_HH-mm-ss").ps1"
Può essere utile anche per fare un semplice gestione del versioning o per backup.
Vedi anche Generatore di istruzioni Add-PnPContentType per site content type SharePoint
Installazione modulo
Per installare il modulo PnP usa Install-ModulePowerShell: Install
# preferibile: multi piattaforma solo per online https://www.toddklindt.com/blog/Lists/Posts/Post.aspx?ID=883
Install-Module -Name PnP.PowerShell
# oppure in alternativa
# SharePoint Online
Install-Module SharePointPnPPowerShellOnline
# SharePoint 2019
Install-Module SharePointPnPPowerShell2019
# SharePoint 2016
Install-Module SharePointPnPPowerShell2016
# SharePoint 2013
Install-Module SharePointPnPPowerShell2013