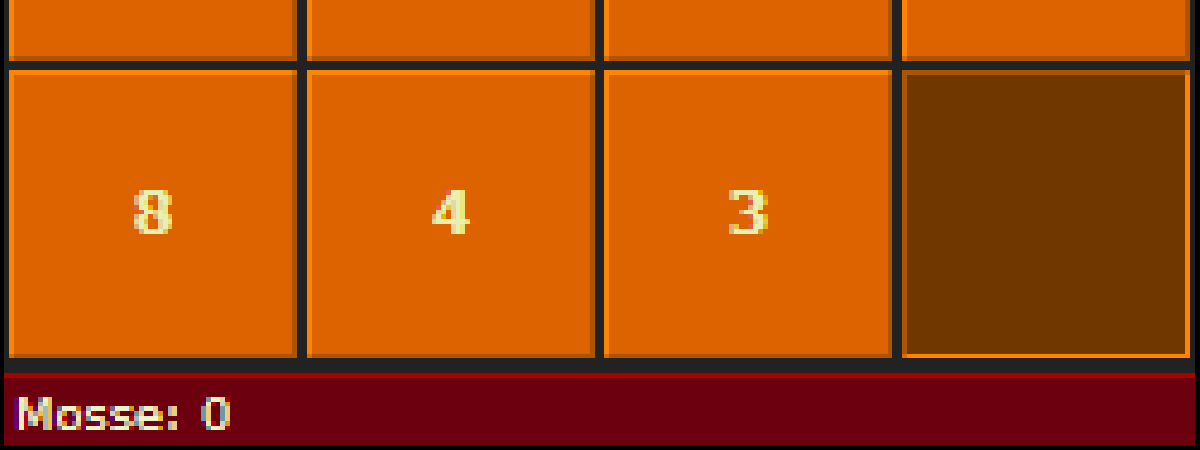
Gioco del 15
Si tratta della versione online del classico Gioco del 15 realizzato in JavaScript.
Lo scopo del gioco è semplice, si tratta di far scorrere i numeri fino a che non sono completamente ordinati da 1 a15.
Il numero 1 deve essere il primo in alto a sinistra, seguito a destra dal numero 2, 3 e 4. Il numero 5 va nella prima casella a sinistra della seconda riga e così via fino al numero 15.
Per spostare le tessere, clicca su uno dei numeri adiacenti alla casella vuota.
Per ricominciare con una nuova sequenza casuale, ricarica la pagina.
Il codice html e JavaScript è il seguente:
Lo scopo del gioco è semplice, si tratta di far scorrere i numeri fino a che non sono completamente ordinati da 1 a15.
Il numero 1 deve essere il primo in alto a sinistra, seguito a destra dal numero 2, 3 e 4. Il numero 5 va nella prima casella a sinistra della seconda riga e così via fino al numero 15.
Per spostare le tessere, clicca su uno dei numeri adiacenti alla casella vuota.
Il codice html e JavaScript è il seguente:
HTML
<div id="sgart15wrapper" class="game15">
<div id="sgart15Game"></div>
<div id="sgart15Status"></div>
<div id="sgart15New" style="display:none"></div>
</div>
JavaScript
var sgart15num = new Array(1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,0);
var sgart15x = new Array(0,1,2,3,0,1,2,3,0,1,2,3,0,1,2,3,0);
var sgart15y = new Array(0,0,0,0,1,1,1,1,2,2,2,2,3,3,3,3,0);
var sgart15dim = 30;
var sgart15game = document.getElementById("sgart15Game");
var sgart15count = 0;
sgart15();
function sgart15(){
sgart15AddCss();
sgart15Random();
sgart15Field();
sgart15Reposition();
}
//sposta casualmente i numeri
function sgart15Random(){
sgart15num = new Array(1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,0);
var defaultSheet = document.styleSheets[0];
for(var i = 0; i < 16; i++){
var p1 = sgart15Rnd(15);
var p2 = sgart15Rnd(15);
//swap
var tmp = sgart15num[p1];
sgart15num[p1] = sgart15num[p2];
sgart15num[p2] = tmp;
}
sgart15count = -1;
}
function sgart15Rnd(max){
return Math.floor(Math.random() * max);
}
//genera il campo di gioco
function sgart15Field(){
var s = "";
for(var i = 0; i < 16; i++){
var n = sgart15num[i];
var t = n;
if (n == 0) {
s += "<div id=\"cell_" + n + "\" class=\"cell0\"> </div>";
} else {
s += "<div id=\"cell_" + n + "\" class=\"cell\">" + n + "</div>";
}
}
sgart15game.innerHTML = s;
sgart15resetCounter();
}
//riposiziona le pedine
function sgart15Reposition(){
var p = sgart15FindOffset(sgart15game);
xOffset = p[0]+1;
yOffset = p[1]+1;
for(var i = 0; i < 16; i++){
var n = sgart15num[i];
var element = document.getElementById("cell_" + sgart15num[i]);
element.style.left = (xOffset + sgart15x[i] * (sgart15dim + 2)) + "px";
element.style.top = (yOffset + sgart15y[i] * (sgart15dim + 2)) + "px";
//aggiunge l'evento onclick
if(n > 0){
element.onclick = sgart15MoveNumber;
if (element.captureEvents) element.captureEvents(Event.CLICK);
}
}
}
//trova la posizione del numero
function sgart15FindPosition(n){
for(var i = 0; i < 16; i++){
if (sgart15num[i] == n){
return i;
}
}
return -1;
}
function sgart15FindOffset(obj) {
var curleft = curtop = 0;
if (obj.offsetParent) {
curleft = obj.offsetLeft
curtop = obj.offsetTop
while (obj = obj.offsetParent) {
curleft += obj.offsetLeft
curtop += obj.offsetTop
}
}
return [curleft,curtop];
}
//controlla se sono in sequenza
function sgart15checkSequence(){
sgart15num
for(var i = 0; i < 15;i++){
if (sgart15num[i] != i+1)
return false;
}
return true;
}
//evento di spostamento
function sgart15MoveNumber(e){
var element;
if (!e) var e = window.event;
if (e.target) element = e.target;
else if (e.srcElement) element = e.srcElement;
if (element.nodeType == 3) // defeat Safari bug
element = element.parentNode;
var n = (element.textContent) ? element.textContent: element.innerText;
var p1 = sgart15FindPosition(n);
if (n <= 0){
return;
}
//trova la posizione libera
var p2 = -1;
var move = false;
if (p1 >= 0 && p1 <= 16){
var x = sgart15x[p1];
var y = sgart15y[p1];
p2 = (x-1)+y*4;
if (x > 0 && sgart15num[p2] == 0){
move = true;
} else {
p2 = (x+1)+y*4;
if (x < 3 && sgart15num[p2] == 0){
move = true;;
} else {
p2 =x+(y-1)*4;
if (y > 0 && sgart15num[p2] == 0){
move = true;
} else {
p2 =x+(y+1)*4;
if (y < 3 && sgart15num[p2] == 0){
move = true;
}
}
}
}
if (move == true){
var tmp = sgart15num[p1];
sgart15num[p1] = sgart15num[p2];
sgart15num[p2] = tmp;
sgart15Reposition();
sgart15updateCounter();
if (sgart15checkSequence()) alert("fine");
}
}
}
function sgart15updateCounter(){
var status = document.getElementById("sgart15Status");
sgart15count++;
status.innerHTML = "Mosse: " + sgart15count;
}
function sgart15resetCounter(){
sgart15count = -1;
sgart15updateCounter();
}
//gestione CSS
function sgart15AddCss() {
var styles = new Array(
new Array("div.game15", "{font-family: Verdana;display: block;overflow: "
+ "hidden;width: 128px; border: 1px solid #000000; padding:0; margin:0px; }" )
, new Array("#sgart15Game" , "{height: 128px; padding: 0; margin: 0; }" )
, new Array("#sgart15Status", " {height: 15px; padding: 0; margin: 0; border-top: 1px solid #AA0000;"
+ "color: #F0F4CC; background-color: #6C000E; font-size: 10px; line-height: 14px;"
+ "text-align: left; vertical-align: middle; padding-left: 2px;}")
, new Array("div.game15 .cell", " {display: block; width:30px; height:30px;"
+ "border-top: 1px solid #FF8500; border-left: 1px solid #FF8500; "
+ "border-right: 1px solid #AC5500; border-bottom: 1px solid #AC5500;"
+ "background-color: #DD6300; vertical-align: middle; text-align:center;"
+ "position: absolute; padding: 0; margin:0px; cursor: pointer; line-height:28px;"
+ "font-size: 12px; font-weight: bold; color: #E8EEB3;}")
, new Array("div.game15 .cell0", " {display: block; width:30px; height:30px;"
+ "border-top: 1px solid #FF8500; border-left: 1px solid #FF8500; "
+ "border-right: 1px solid #AC5500; border-bottom: 1px solid #AC5500;"
+ "background-color: #DD6300; vertical-align: middle; text-align:center;"
+ "position: absolute; padding: 0; margin:0px; cursor: pointer; line-height:28px;"
+ "font-size: 12px; font-weight: bold; color: #E8EEB3;}")
, new Array("div.game15 .cell0", "{border-top: 1px solid #AC5500; border-left: 1px solid #AC5500;"
+ "border-right: 1px solid #FF8500; border-bottom: 1px solid #FF8500; "
+ "background-color: #703700;}")
, new Array("div.game15 .cell:hover", " {background-color: #C85900; color: #FFFFFF;}")
);
var s = "";
if(document.styleSheets[0].insertRule){
for(var i = styles.length - 1; i >= 0; i--)
document.styleSheets[0].insertRule(styles[i][0] + styles[i][1], 1);
}else{
for(var i = 0; i < styles.length; i++)
document.styleSheets[0].addRule(styles[i][0], styles[i][1]);
}
}
function sgart15GetRules() {
if (!document.styleSheets) return;
var theRules = new Array();
if (document.styleSheets[0])
theRules = document.styleSheets[0].cssRules
else if (document.styleSheets[0].rules)
theRules = document.styleSheets[0].rules
else return;
return theRules;
}