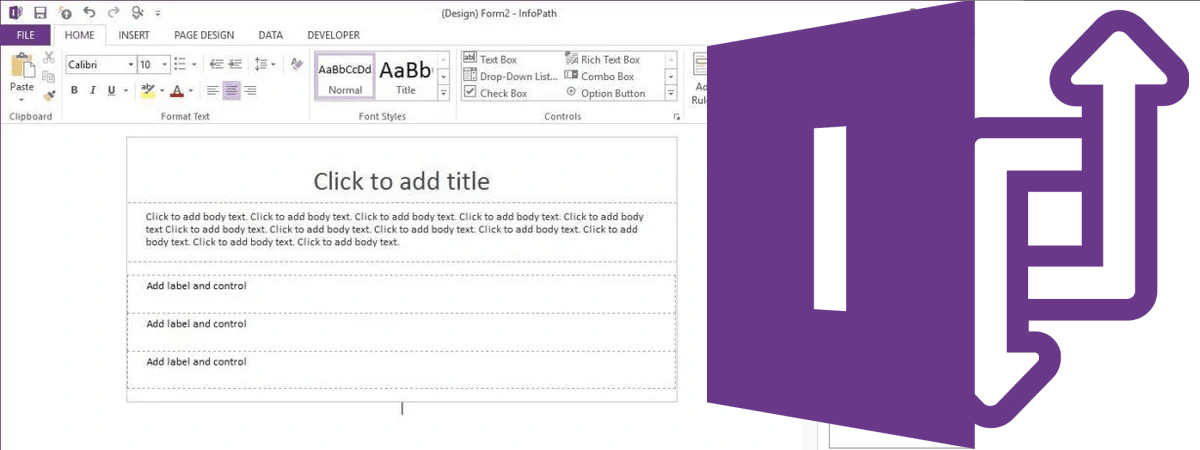
InfoPath: lettura xml
La seguente classe torna utile per leggere i valori memorizzati in un form InfoPath 2007 salvato in una form library di SharePoint 2007 (MOSS).
In pratica, passandogli un file xml che rappresenta il form InfoPath (ovvero quello che viene salvato in una form library), rileva automaticamente i namespace utilizzati ed espone dei metodi per leggere le proprietà dei campi (nodi xml).
In pratica, passandogli un file xml che rappresenta il form InfoPath (ovvero quello che viene salvato in una form library), rileva automaticamente i namespace utilizzati ed espone dei metodi per leggere le proprietà dei campi (nodi xml).
C#
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Xml;
public class FormXSN
{
private XmlNamespaceManager _xns;
private XmlDocument _form;
public FormXSN(XmlDocument formXsn)
{
this._form = formXsn;
//add namespaces read from xml
_xns = new XmlNamespaceManager(_form.NameTable);
//"dfs", "http://schemas.microsoft.com/office/infopath/2003/dataFormSolution"
foreach (XmlAttribute nsAttr in Form.DocumentElement.Attributes)
{
if (nsAttr.Prefix == "xmlns")
_xns.AddNamespace(nsAttr.LocalName, nsAttr.Value);
}
//"my", "http://schemas.microsoft.com/office/infopath/2003/myXSD/yyyy-MM-ddThh:mm:ss"
//the last part yyyy-MM-ddThh:mm:ss change on every form
foreach (XmlNode node in Form.DocumentElement.ChildNodes)
{
if (node.NodeType.Equals(XmlNodeType.Element) == true && _xns.HasNamespace(node.Prefix) == false)
{
foreach (XmlAttribute nsAttr in node.Attributes)
{
if (nsAttr.Prefix == "xmlns")
_xns.AddNamespace(nsAttr.LocalName, nsAttr.Value);
}
}
}
}
public XmlDocument Form
{
get { return _form; }
set { _form = value; }
}
public XmlNamespaceManager NameSpaceManager
{
get { return _xns; }
}
//nodeName: the name of the field in form
public XmlNode SelectSingleNode(string nodeName)
{
return Form.SelectSingleNode(string.Format("//my:{0}", nodeName), NameSpaceManager);
}
public string SelectSingleNodeText(string nodeName)
{
return Form.SelectSingleNode(string.Format("//my:{0}", nodeName), NameSpaceManager).InnerText;
}
}